Exploring OOP Programming: Concepts and Applications
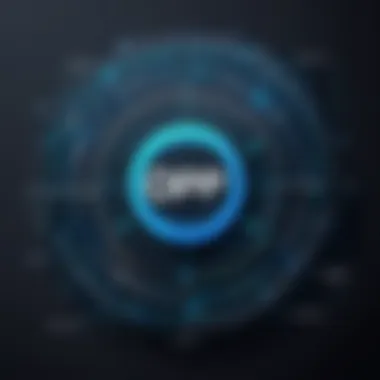
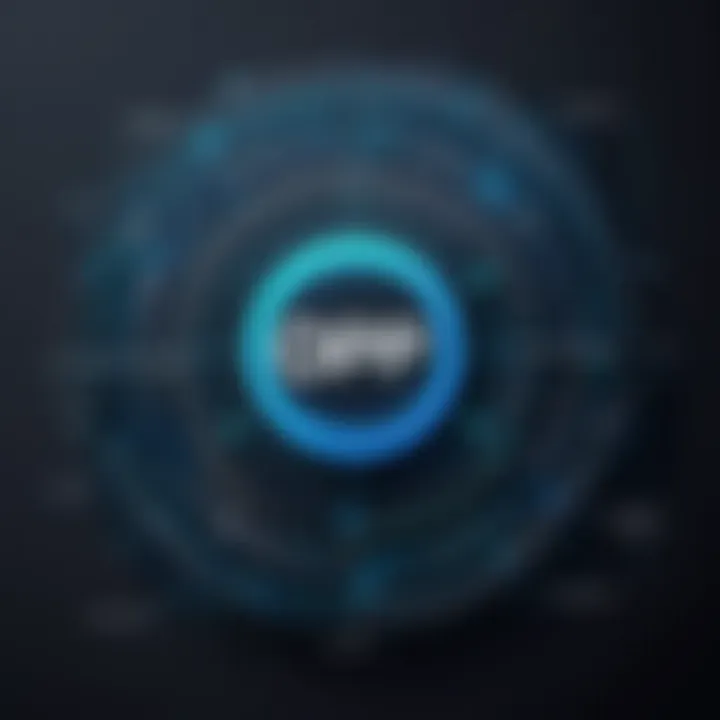
Intro
Object-Oriented Programming (OOP) is a programming paradigm that emphasizes the use of objects and classes. This approach allows developers to design software in a more modular and organized way. In the context of information technology, OOP is increasingly important. It allows for easier maintenance, scalability, and reuse of code. As IT professionals navigate complex systems, understanding OOP becomes a vital part of their skill set.
In this article, we will explore the core concepts of OOP. We will also discuss its applications and best practices. Our goal is to equip readers, especially tech enthusiasts and developers, with the insights needed to effectively implement OOP in various projects.
Intro to Oops Programming
Object-Oriented Programming (OOP) represents a paradigm that profoundly influences the way software development is approached. As the technology landscape evolves, understanding OOP becomes crucial for IT professionals and developers. The principles of OOP not only help in structuring code more effectively but also facilitate problem-solving in a more intuitive manner. This article aims to unpack OOP, providing insights into its fundamental concepts and practical applications.
The significance of OOP extends beyond mere coding practices. It promotes concepts such as reusability, scalability, and maintainability, which are essential for modern software development. Through OOP, developers can create systems that are not only easier to manage but also align with real-world processes.
This section sets the groundwork for a deeper discussion about what OOP is and how it has evolved over time. By understanding the core definition and historical context of OOP, readers can appreciate its impact on software engineering.
Definition of OOP
Object-Oriented Programming is a programming methodology that utilizes "objects" to design applications and computer programs. Objects can encapsulate data and functions, which enables the developer to model complex systems more naturally.
At its core, OOP revolves around four main principles: encapsulation, inheritance, polymorphism, and abstraction. These principles are not just theoretical; they serve practical purposes in crafting robust and scalable applications. With encapsulation, for instance, data hiding ensures that internal states of objects are shielded from the outside world, promoting security and modularity.
OOP allows programmers to create code that mimics interactions in the real world, which can lead to more intuitive designs. This method aids in building software that is easier to understand and maintain over time, creating a significant advantage in competitive programming environments.
History and Evolution
The evolution of Object-Oriented Programming traces back to the 1960s. The early days saw languages like Simula, which introduced the concept of classes and objects. However, it was not until the emergence of languages such as Smalltalk and C++ that OOP gained broader acceptance. These languages laid the foundation for many of the principles and practices that are now standard in programming education.
In the late 1980s and early 1990s, OOP began to permeate mainstream programming through various languages like Java, C#, and Python. Each of these languages brought its interpretations and enhancements to the paradigms established by earlier models. The transition from procedural programming to OOP brought a paradigm shift in how programmers approached problem-solving and application design.
Over the years, the popularity of OOP has been driven by the need for reusable and maintainable code in increasingly complex systems. The introduction of modern frameworks and tools has further solidified the use of OOP in the software development lifecycle.
"OOP is not just another fad in programming; it has become a fundamental approach to building software that enforces good design principles."
As we move forward, the principles derived from OOP will likely continue to adapt, responding to the needs of developers and the demands of new technologies while retaining their core values. For IT professionals, understanding OOP not only enhances their programming skills but also prepares them for the future landscape of software development.
Fundamental Concepts of Oops
Understanding the fundamental concepts of Object-Oriented Programming (OOP) is essential for both novice and experienced developers. These concepts are the building blocks that underpin the OOP paradigm, providing a framework for structuring and organizing code efficiently. Emphasizing classes, objects, encapsulation, inheritance, and polymorphism allows developers to create systems that are not only robust but also scalable and maintainable. This article aims to dissect these core principles in detail, highlighting their significance and practical applications.
Classes and Objects
Classes serve as blueprints for creating objects in OOP. An object is an instance of a class that encapsulates data and behaviors. The primary benefit of using classes is their ability to model real-world entities. For instance, consider a class called . This class can define properties like , , and , as well as methods such as and .
By creating different objects from the class, developers can represent individual cars with specific attributes. This modularity simplifies code management and enhances readability. When working with classes and objects, it âs important to understand the relationships and interactions between various objects, particularly how they can communicate with one another. This leads to cleaner, organized, and efficient coding practices.
Encapsulation
Encapsulation is a core principle of OOP that promotes data hiding and abstraction. It involves bundling the data (attributes) and methods (functions or procedures) that operate on the data into a single unit called a class. This concept ensures that the internal state of an object cannot be accessed directly from outside its class; it can only be manipulated through specified methods, known as accessors and mutators.
The primary advantage of encapsulation is enhanced security. Sensitive data is protected from unauthorized access. Additionally, it simplifies maintenance and modification. If the internal workings of a class change, as long as the public interface remains the same, the external code remains unaffected. This characteristic raises the quality of software development by minimizing bugs and improving code clarity.
Inheritance
Inheritance allows a new class, known as a subclass, to inherit characteristics and behaviors from an existing class, called a superclass. This mechanism promotes code reusability, reducing redundancy and pursuing a more efficient coding structure. For example, if a class called exists, subclasses such as , , and can derive properties and methods from it, like and .
Inherited classes can introduce additional features or override existing methods to fit their specific needs. However, it is crucial to manage inheritance carefully. Overusing inheritance can lead to complex hierarchies that are difficult to understand. Favoring composition over inheritance can sometimes yield a more flexible design.
Polymorphism
Polymorphism refers to the ability of different classes to respond to the same method call in a manner appropriate to their type. This capability can be achieved through method overriding and overloading. Method overriding applies when a subclass provides a specific implementation of a method that is already defined in its superclass. In contrast, method overloading allows multiple methods with the same name to coexist in the same class, differentiated by their parameter list.
The main benefit of polymorphism is that it supports the dynamic behavior of methods. This characteristic enhances code flexibility and extensibility, allowing developers to implement changes efficiently. With polymorphism, software systems can grow without significant restructuring, making them more adaptive to future requirements.
"The essence of OOP is solving problems through the systematic modeling of real-world systems."
Understanding these fundamental concepts of OOP is critical not only for the development of complex software systems but also for fostering a culture of collaborative coding. As developers explore and implement these principles, they gain insights into creating clean, efficient, and maintainable code. This ongoing learning process ensures that OOP remains a relevant and powerful paradigm in modern software development.
Principles of Oop Design
Understanding the principles of object-oriented programming design is essential for any software developer. These principles not only guide developers in creating robust, maintainable solutions but also enhance collaboration among team members. By adhering to these principles, developers can ensure that their code is organized, efficient, and scalable.
The foundational principles often referenced in OOP design encompass SOLID, DRY, and KISS. Each of these plays a significant role in addressing common challenges in software development, such as code complexity and maintainability.
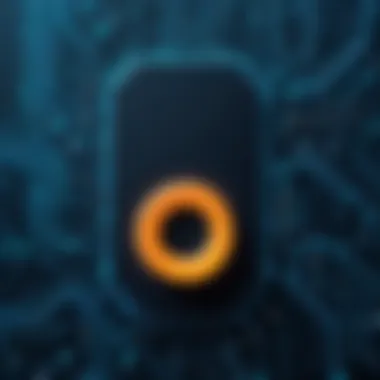
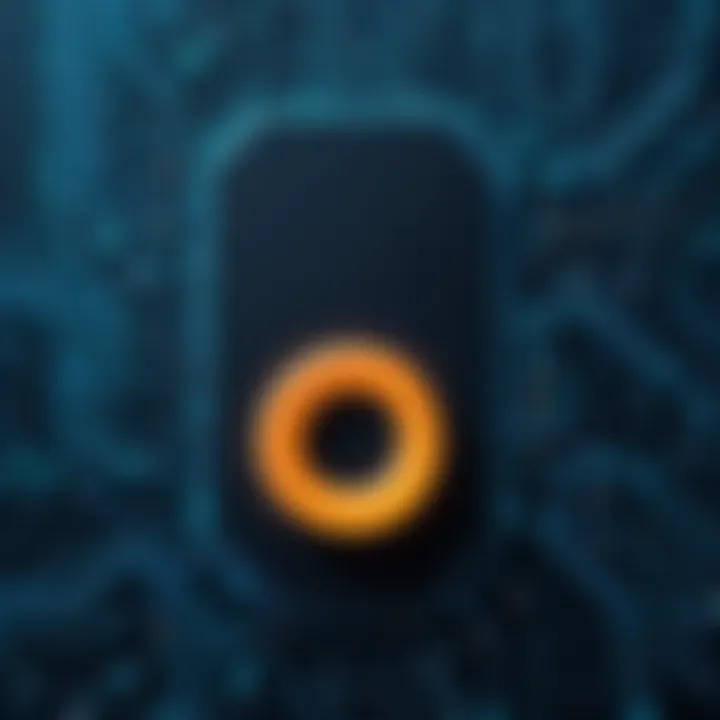
SOLID Principles
The SOLID principles form a set of five guidelines that aim to make software designs more understandable, flexible, and maintainable. Hereâs a brief exploration of each principle:
- Single Responsibility Principle: A class should only have one reason to change, meaning it should only have one job or responsibility. This reduces the impact of changes, minimizing the risk of unintended consequences.
- Open/Closed Principle: Software entities should be open for extension but closed for modification. This encourages developers to extend existing code rather than altering it, which fosters stability.
- Liskov Substitution Principle: Subtypes must be substitutable for their base types without altering the correctness of the program. This principle ensures that a derived class can stand in for a base class without causing issues.
- Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use. This means that decoupling interfaces can lead to better design by keeping them small and focused.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules. Both should depend on abstractions, which helps in reducing the coupling between different classes.
By implementing these principles, developers can prevent tight coupling and ensure their code remains manageable as projects grow.
DRY Principle
DRY stands for Donât Repeat Yourself. This principle emphasizes the importance of reducing the duplication of code. Repeated code can lead to inconsistencies and makes maintenance challenging. When a change is required, developers need to track down all duplicated code and modify it, increasing the risk of oversight.
To implement the DRY principle effectively:
- Use functions or methods to encapsulate repetitive logic.
- Leverage inheritance to share common behavior among classes.
- Apply design patterns that promote reusability, such as the Factory or Strategy patterns.
By embracing this principle, developers can create cleaner and more efficient code.
KISS Principle
KISS stands for Keep It Simple, Stupid. This design principle asserts that simplicity should be a key goal in design. Complex solutions can lead to more bugs and make code harder to maintain. Therefore, developers should strive for designs that are as straightforward as possible.
To apply the KISS principle, consider the following:
- Avoid over-engineering solutions; simplicity often yields the best results.
- Use clear and concise naming conventions for classes and methods, improving code legibility.
- Break down problems into smaller, more manageable pieces.
"Simplicity is the ultimate sophistication." - Leonardo da Vinci
By keeping code simple and concise, developers can focus on functionality without getting bogged down by unnecessary complexity.
In summary, the principles of OOP designâSOLID, DRY, and KISSâprovide a framework that enhances software development efficacy. By applying these principles, IT professionals can create systems that are not only effective but also easier to maintain and extend in the long run.
Common Design Patterns in Oops
Design patterns are proven solutions to common problems that arise in software development. In the context of Object-Oriented Programming (OOP), design patterns help to streamline the development process by providing a clear structure that developers can follow. There are three main types of design patterns: creational, structural, and behavioral. Each type addresses specific challenges within software engineering. Understanding and implementing these patterns enhances code maintainability, scalability, and readability. This section delves into the three categories of design patterns and their significance in OOP.
Creational Patterns
Creational design patterns focus on object creation mechanisms, aiming to create objects in a manner suitable to the situation. Rather than instantiating classes directly, these patterns provide solutions to create instances more flexibly and efficiently.
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access. This is useful when a single point of control is needed, such as in configuration settings.
- Factory Pattern: Uses methods to create objects without specifying the exact class of the object that will be created. This decouples object creation from its usage, leading to higher flexibility.
- Builder Pattern: Allows for the step-by-step construction of a complex object. It separates the construction process from the representation to create different representations of an object.
Using these creational patterns results in a system that is much easier to understand and maintain.
Structural Patterns
Structural design patterns ease the composition of classes and objects to form larger structures. These patterns help ensure that if one part of a system changes, the entire system doesn't need to start over.
- Adapter Pattern: Acts as a bridge between two incompatible interfaces. It allows classes to work together that could not otherwise because of incompatible interfaces.
- Decorator Pattern: Adds new functionality to an existing object dynamically without altering its structure. It promotes single responsibility by allowing for responsibilities to be divided into classes.
- Composite Pattern: Enables you to compose objects into tree structures to represent part-whole hierarchies. It makes it easier to work with complex tree structures.
Structural patterns facilitate better code organization and improve code adaptation in an evolving codebase.
Behavioral Patterns
Behavioral design patterns focus on improving or streamlining the communication between disparate objects. They provide a means to define how objects interact in a flexible manner.
- Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes its state, all its dependents are notified and updated automatically. This is widely used in event handling systems.
- Strategy Pattern: Enables selecting an algorithm's behavior at runtime. It defines a family of algorithms, encapsulates each one, and makes them interchangeable.
- Command Pattern: Encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations.
These behavioral patterns contribute to more efficient communication among objects and improve the overall design of software systems.
Design patterns, when applied properly, can drastically reduce the complexity of your code and improve its maintainability.
In summary, an in-depth understanding of common design patterns in OOP is vital for any developer looking to enhance their software development skills. By integrating creational, structural, and behavioral patterns into their processes, IT professionals can develop systems that are robust and flexible.
Applications of Oops in Software Development
Understanding the applications of Object-Oriented Programming (OOP) is vital for any IT professional. OOP transforms the way software is developed, making it more efficient, modular, and easier to maintain. This section will explore how OOP serves different sectors within software development. The focus will be on web development, mobile app development, and game development. Each area utilizes OOP principles differently, yet they all benefit from its core concepts.
OOP in Web Development
In web development, OOP enhances scalability and reduces complexity. Frameworks such as Django and Ruby on Rails utilize OOP principles to promote rapid application development. The structure provided by classes and objects allows developers to create modular pieces of code. These can be reused across various projects, saving time and effort. Moreover, OOP helps manage large codebases effectively, which is crucial for maintaining websites that grow over time.
Benefits of OOP in web development include:

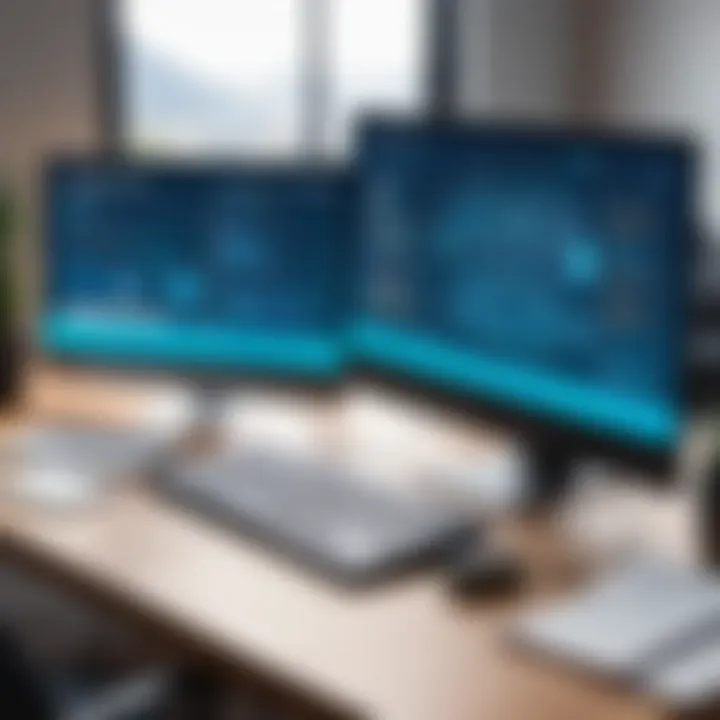
- Improved organization: Code is easier to navigate when encapsulated in classes.
- Enhanced collaboration: Multiple developers can work on different components without conflicts.
- Faster debugging: Errors are easier to locate in a well-structured OOP codebase.
OOP also supports design patterns such as MVC (Model-View-Controller), which separate the application logic from user interface components. This separation enhances code maintainability and scalability.
OOP in Mobile App Development
Mobile app development has seen significant advancements through OOP. Frameworks like Android and iOS SDK leverage OOP principles to create responsive applications. They provide well-defined structures for organizing code, which allows for quick iterations and updates. The encapsulation of data and methods in classes helps build intuitive user experiences.
Key advantages of using OOP in mobile app development include:
- Code reusability: Developers can use existing classes in new applications, reducing redundancy.
- Improved user experiences: OOP allows for the easy implementation of complex features without impacting overall performance.
- Efficient memory management: OOP can lead to better resource utilization, which is critical for mobile devices with limited memory.
Mobile frameworks often adopt OOP patterns that encourage clean architecture, enhancing long-term maintainability while managing the complexity of developing mobile applications.
OOP in Game Development
Game development is another area where OOP shines. Games often involve multiple entities that interact in complex ways, making OOP particularly useful. Using OOP, developers can create abstract classes and inherit them to create different game objects like characters, weapons, and environments.
The benefits of OOP in game development include:
- Natural representation of objects: Real-world entities can be represented as objects, making it easier for developers to conceptualize.
- Flexibility in design: The ability to modify properties of objects quickly allows developers to adapt to changes without significant overhauls.
- Efficient resource management: OOP can optimize how game resources are loaded and modified, leading to better performance.
Game engines like Unity and Unreal Engine heavily rely on OOP to structure game mechanics and behaviors. This reliance enables developers to create complex games with rich interactions in manageable steps.
By employing Object-Oriented Programming, software developers can enhance not only performance but also efficiency in collaborative environments, leading to robust and scalable applications.
Advantages of Oops Programming
Understanding the advantages of Object-Oriented Programming (OOP) is essential for professionals involved in software development. OOP offers various benefits that streamline processes and enhance code quality. These advantages highlight why OOP remains a cornerstone of modern programming practices.
Code Reusability
One of the key advantages of OOP is code reusability. In traditional programming paradigms, developers often write the same code multiple times for similar functionalities. This can lead to larger codebases and increased chances of errors. With OOP, developers can create classes that encapsulate properties and behaviors. Once a class is defined, it can be reused across multiple programs without the need to rewrite code. This not only saves time but also contributes to consistency throughout the project.
Moreover, inheritance allows new classes to extend existing ones, reusing code while adding or modifying functionalities. This layered approach promotes a modular design, making it easier to adapt or expand software applications over time.
Scalability
Scalability is another significant benefit of OOP. As projects grow, maintaining command over complex code can become challenging. OOP provides a structured framework that allows developers to scale their applications effectively. By utilizing principles such as encapsulation, new features or changes can be introduced with minimal disruption to existing code.
For instance, in web development, frameworks built on OOP principles, like Django or Ruby on Rails, enable developers to manage large applications by breaking them into smaller, manageable components. This division into classes and objects aids developers in maintaining and scaling projects without burdening performance.
Maintainability
Maintainability is crucial in software development, given the ever-changing technological landscape. OOP facilitates easier maintenance through its design principles. The separation of concerns allows developers to work on individual classes without affecting others. This means that if a bug arises, it can be identified and fixed in a specific area of the code.
OOP promotes a holistic approach to software development, making it essential for modern practices.
In summary, the advantages of OOP programming, particularly in terms of code reusability, scalability, and maintainability, are significant. They streamline processes and improve the overall quality of software applications, which makes it indispensable for today's developers.
Challenges in Implementing Oops
In Object-Oriented Programming, various complexities arise that can hinder implementation. Recognizing these challenges is essential for IT professionals aiming to leverage OOP effectively. The difficulties include managing complexity, coping with abstraction overhead, and ensuring team coordination. Addressing these challenges improves software quality, enhances team productivity, and streamlines development processes.
Complexity Management
Managing complexity is a significant challenge in OOP. As systems become large and intricate, understanding relationships between classes and objects can become difficult. Developers must ensure that the system remains comprehensible. To manage complexity, it is essential to adopt a modular design approach. By breaking down the system into smaller, manageable components, it becomes easier to maintain and understand the overall structure. Additionally, unified documentation can play a vital role in simplifying complexity. Regular reviews, code refactoring, and strict adherence to design patterns will help coders to tackle these issues effectively.
"A well-structured OOP system reduces cognitive load, facilitating better collaboration and maintenance."
Overhead of Abstraction
Abstraction is a fundamental concept in OOP that enhances code reusability. However, it also introduces some overhead. Abstraction layers can lead to performance inefficiencies if not managed correctly. Developers may face challenges in determining the right level of abstraction. Striking a balance between too much and too little abstraction is crucial. Excessive abstraction can complicate simple tasks, while insufficient abstraction might result in code duplication. Testing the design for performance impacts is important to ensure that the abstraction serves its intended purpose without degrading system performance.
Team Coordination
Implementation of OOP relies on effective team coordination. When various team members work on different components of the same system, discrepancies may arise. Clear communication is paramount to ensure everyone understands the design architecture. Regular meetings and sessions can help align team members with the system goals. Version control tools, like Git, facilitate collaboration by allowing teams to track changes efficiently. Establishing a robust code review process ensures that all team contributions align with the overall design principles and best practices of OOP.
Modern Tools and Frameworks for Oops
The landscape of software development is continually evolving. As more developers embrace Object-Oriented Programming (OOP) methodologies, the reliance on modern tools and frameworks has become paramount. These tools not only streamline the development process but also enhance productivity and code quality. The right framework or programming language can significantly influence a developerâs effectiveness and the resulting softwareâs performance.
Popular Programming Languages
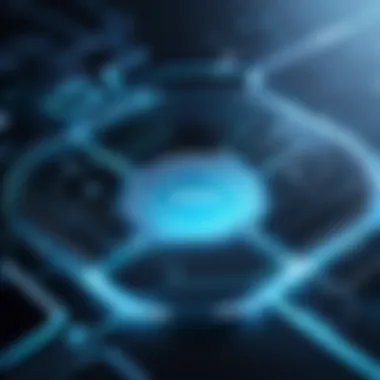
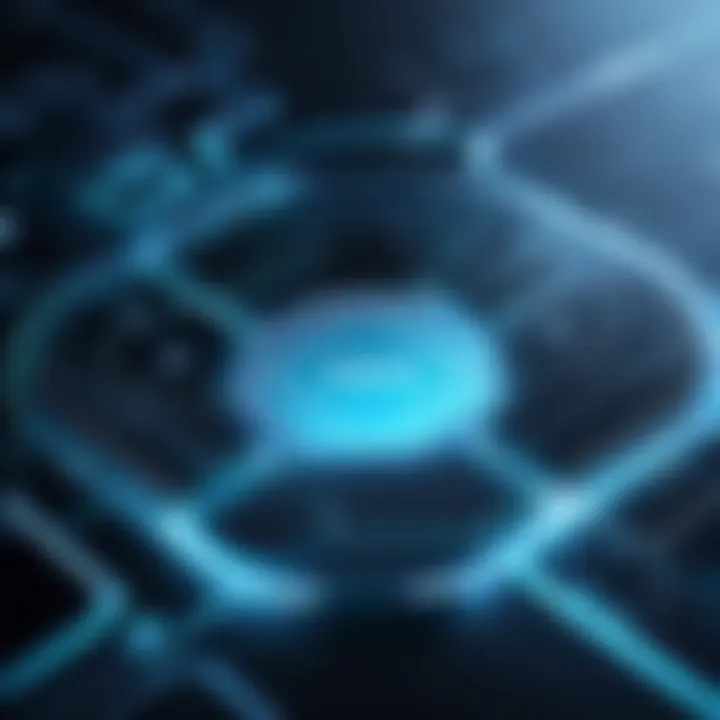
Several programming languages are designed around OOP principles. These languages provide support for key concepts like classes, objects, and polymorphism. Here are some popular ones:
- Java: Known for its portability across platforms due to the Java Virtual Machine (JVM), Java is widely used in enterprise applications. Its strong typing and extensive libraries make it suitable for complex OOP projects.
- Python: This language emphasizes simplicity and readability. Python's dynamic typing and extensive libraries facilitate rapid application development. Itâs popular for both web development and data science, leveraging OOP concepts effectively.
- C++: A powerful language that extends the capabilities of C with object-oriented features. C++ is used frequently in system/software development, allowing control over system resources while employing OOP.
- Ruby: Designed for simplicity and productivity, Ruby uses OOP extensively. Its framework, Ruby on Rails, is renowned for building web applications quickly and efficiently.
Choosing the appropriate language depends on the specific requirements of a project, developer preferences, and existing infrastructure. Each of these languages brings its own set of advantages and considerations.
Integrated Development Environments
An Integrated Development Environment (IDE) is crucial for enhancing the productivity of developers working with OOP. IDEs provide tools for writing, testing, and debugging code in a single workspace. Common features include syntax highlighting, code completion, and integrated debugging tools. Some renowned IDEs include:
- Eclipse: Primarily used for Java, it offers great support for other languages through plugins.
- Visual Studio: Esteemed for C# and C++, it includes powerful debugging capabilities that streamline development.
- PyCharm: Specifically designed for Python, it enhances productivity with its smart code completion and project navigation.
Using a good IDE can reduce development time and facilitate collaboration among team members. As OOP projects become more complex, these environments provide essential support.
Frameworks and Libraries
Frameworks and libraries play significant roles in accelerating OOP development. They offer pre-built components that handle common tasks, allowing developers to focus on the unique aspects of their applications. Some notable frameworks and libraries include:
- Spring: A framework for Java that provides a comprehensive infrastructure for developing Java applications. It supports various features such as dependency injection and aspect-oriented programming.
- Django: A high-level Python web framework that encourages rapid development. Django follows the
Best Practices in Oops Programming
Best practices in OOP programming are essential for maintaining an efficient and manageable codebase. These practices provide a reliable framework for developers, ensuring that their code is both flexible and scalable. Implementing best practices can significantly reduce development time and improve collaboration among team members. By applying proven strategies, developers can minimize common pitfalls associated with OOP principles, enhancing productivity and software quality.
Effective Use of Inheritance
Inheritance is a powerful feature of OOP that allows a class to inherit properties and behaviors from another class. However, its effective use requires careful planning. Developers should focus on creating a clear hierarchy among classes. A deep inheritance tree can lead to complex interdependencies, making the code harder to manage. Instead, prefer a shallow hierarchy and compose objects where necessary. This prevents fragile base class problems, where changes to the base class can inadvertently affect derived classes.
Additionally, always favor composition over inheritance when appropriate. In many cases, using composition can lead to more flexible and easier-to-understand designs. This approach allows different behaviors to be mixed and matched on an object without tight coupling.
Documentation and Code Commenting
Documentation and code commenting are crucial aspects of any software development process. Clear comments within the code help other developers (and future you) understand the rationale behind certain decisions.
- Use comments to explain why a piece of code exists, not just what it does.
- Avoid obvious comments that do not add value.
- Maintain an up-to-date README file to guide users and other developers through your project.
In addition to comments, formal documentation should be created for significant components. Tools like Javadoc can automate this process for Java projects. Good documentation not only aids maintainability but also helps onboard new team members quickly.
Testing OOP Code
Testing in OOP is vital for ensuring the robustness of the system. Each class should have its own set of unit tests that verify its behavior in isolation. Use frameworks such as JUnit for Java or pytest for Python to facilitate this process. Proper testing encourages you to write clean, modular code. To check that your testing strategy is effective:
- Write tests before writing the code (Test-Driven Development).
- Use mocking frameworks to isolate dependencies in your tests, ensuring that you test only one component at a time.
- Regularly run automated tests to ensure ongoing code integrity when changes are made.
"With the proper testing practices, developers can avoid introducing defects during enhancements or refactoring."
By incorporating these three best practicesâeffective use of inheritance, thorough documentation, and diligent testingâdevelopers can enhance the quality and maintainability of OOP projects, ultimately leading to more successful outcomes in software development.
Epilogue
The conclusion of this article is to provide a solid framework for understanding the essentials of Object-Oriented Programming (OOP). This section emphasizes the relevance of the concepts, applications, and best practices discussed throughout the article. It also highlights the evolution of OOP, which offers critical insights for IT professionals aiming to enhance their software development skills.
One key aspect is the adaptability of OOP methodologies. As technologies evolve, understanding OOP allows developers to embrace new paradigms and tools more effectively. It grants flexibility in programming, thus enabling rapid development cycles and maintaining high levels of code quality.
Another important benefit of OOP is its potential for facilitating collaboration. When projects are built with OOP principles, team members can work on different components independently. This modular approach reduces bottlenecks and promotes efficiency.
Consider also how OOP fosters code reuse. By using inheritance and polymorphism, developers can minimize redundancy and enhance overall productivity. This results in reduced development time and costs, allowing more focus on innovation rather than repetitive coding tasks.
Integrating OOP practices can lead to significant improvements in system performance. Well-structured OOP can enhance maintainability and scalability, critical factors in today's fast-paced tech landscape. As demands change over time, scalability becomes crucial for evolving applications.
In summary, the conclusion emphasizes OOP's enduring value as a programming paradigm and its tangible benefits. By adopting the principles and practices discussed, developers can navigate the complexities of modern software environments with greater ease.
Future Trends in OOP
Looking ahead, several trends are poised to shape the future of OOP. One prominent trend is the integration of Artificial Intelligence into object-oriented systems. As AI technologies progress, there will be an increasing necessity for OOP structures that efficiently handle complex data models and evolving algorithms. This integration will require a fluent understanding of both AI methodologies and OOP principles, merging the two for more efficient software solutions.
Additionally, the rise of microservices architecture will influence the role of OOP. Unlike traditional monolithic applications, microservices will challenge OOP principles to create more lightweight and focused solutions. Developers may need to adapt traditional inheritance and encapsulation concepts to fit within the distributed service environments that microservices promote.
The adoption of functional programming concepts within OOP is also on the horizon. Languages like JavaScript and Python are beginning to merge functional and object-oriented features. This hybrid approach allows developers to take advantage of the benefits offered by both paradigms, enriching their codebase.
Lastly, the emphasis on better tooling and frameworks will enhance OOP's efficiency. Integrated development environments (IDEs) and specialized libraries will continue to streamline coding practices, improving productivity and reducing errors.
Final Thoughts
As we conclude our exploration of Object-Oriented Programming, it is crucial to reflect on its role in the broader context of software development. The principles and practices of OOP provide a robust framework that supports more than just coding but also collaboration and innovation.
OOP is not a static concept; instead, it evolves alongside the technology landscape. This adaptability is a significant asset. As developers encounter new challenges, OOP's principles will continue to provide solutions that promote sustainability and efficiency.
Engaging with OOP means committing to a mindset that values structure, scalability, and maintainability. While adopting these principles may present initial learning curves, mastering them will undoubtedly reap long-term benefits for developers and their organizations.