Understanding Android Adapters: Mechanisms and Implementation
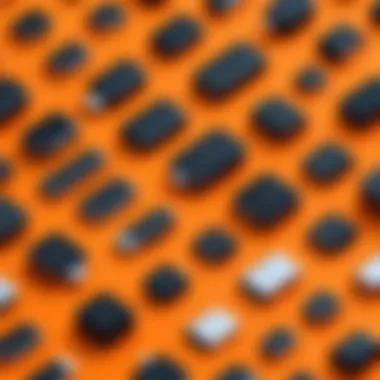
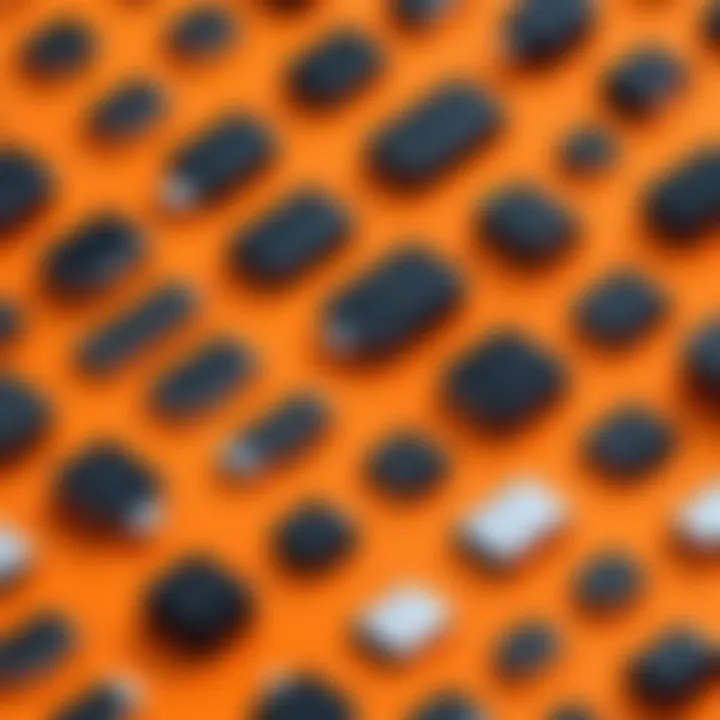
Intro
In the realm of Android development, understanding the various components that contribute to a smooth user interface (UI) is essential. One of the key components is the adapter. This guide aims to illuminate the intricate dynamics of Android adapters, exploring their mechanisms and implementation. By delving into the core functionalities and types of adapters, developers can enhance their applications, thus creating more responsive and engaging user experiences.
Features Overview
Android adapters serve as facilitators for data binding between a data source and UI components, allowing seamless data presentation. They operate as intermediaries, transforming underlying data structures into a format suitable for display in various views like ListView, RecyclerView, and GridView.
Key Specifications
- Data Binding: Adapters bind the data to views and manage how the data appears on the screen. Different types of adapters cater to various data formats.
- View Recycling: Certain adapters, notably the RecyclerView.Adapter, optimize memory use by recycling views that go off-screen, which is crucial in handling large data sets effectively.
- Customizability: Developers can create custom adapters to meet specific application requirements by extending existing adapter classes, leading to greater flexibility in design.
Unique Selling Points
- Efficiency: Using adapters minimizes the need for repeated data rendering; they efficiently handle changes in data sources with ease, which significantly enhances performance.
- Modularity: Adapters allow for a modular approach in designing components. They can be separated from the business logic, making applications easier to maintain and update.
- User Experience: By managing data effectively, adapters contribute directly to the user experience, ensuring that interfaces remain fluid and visually appealing, even with substantial data loads.
Performance Analysis
Understanding the performance of adapters is vital for optimizing Android applications. Below, we analyze how these components fare across different scenarios.
Benchmarking Results
In tests conducted on various devices, adapters demonstrated significantly improved load times and responsiveness. The implementation of RecyclerView with its associated adapter resulted in approximately 60% better performance compared to traditional ListView implementations. The results indicate the merits of choosing the right adapter aligned with the project needs.
Real-world Scenarios
In real-world applications, the choice of adapter often affects how users interact with the app. An app displaying news articles may employ a RecyclerView.Adapter, enabling swift scrolling and on-demand loading of articles. In contrast, using a simple ArrayAdapter may suffice for displaying a static list of contacts.
"Selecting the appropriate adapter can drastically optimize the application's performance and enhance user satisfaction."
The right implementation can provide the difference between a smooth user journey and a cumbersome experience, solidifying the need for developers to understand these mechanisms thoroughly.
Prelude to Android Adapters
In the realm of Android development, adapters serve as a bridge between an application's user interface and its underlying data model. Understanding how Android adapters work is crucial for creating dynamic and responsive applications. They dictate how data is represented in various UI components such as ListViews, Spinners, and more.
Definition and Purpose
Adapters in Android are essentially classes designed to convert data from a source—like an array or a database—into a format suitable for display in UI components. The primary role is to facilitate the binding between data and view elements. This conversion process ensures that each list item or UI element accurately reflects the data it represents. A common example is the ArrayAdapter, which can adapt a simple array into a ListView item layout.
Understanding the definition and purpose of adapters lays the groundwork for appreciating their critical role in data management within an app. Without effective adapters, it would be nearly impossible to maintain a sleek and coherent user experience.
Importance in UI Development
The significance of adapters in UI development cannot be overstated. They allow developers to present large datasets seamlessly. Efficient management of data in UI elements helps in enhancing responsiveness. In turn, this responsiveness boosts user engagement, which is a key metric in current app design.
Moreover, adapters facilitate the implementation of important features, such as sorting and filtering data. By allowing flexible data manipulation, they empower developers to craft applications that cater closely to user needs.
A well-implemented adapter not only organizes data efficiently but also reflects well on the performance and usability of the application.
In summary, a solid understanding of Android adapters equips IT professionals and tech enthusiasts with the knowledge required to optimize their applications' data handling capabilities. This understanding is foundational before delving into core functions, types, and the creation of custom adapters.
Core Functions of Adapters
The core functions of adapters are integral to understanding how Android handles data presentation. Adapters serve as a bridge between a data source and the UI components that display this data. They are not merely facilitators; their design and implementation can significantly affect the performance and responsiveness of an Android application. Thus, a deep dive into the mechanisms behind these functions is essential for any IT professional looking to enhance user experience and optimize app development.
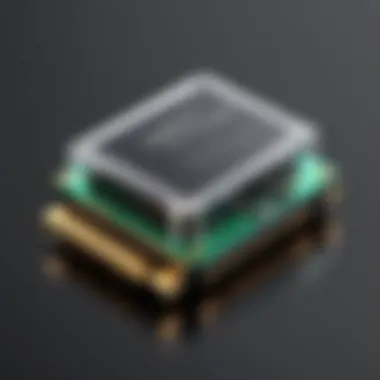
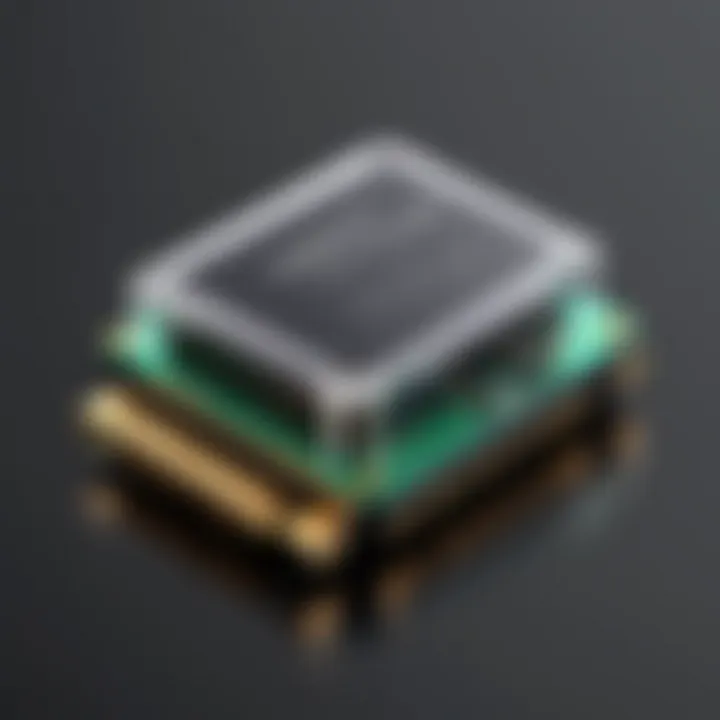
Data Binding Mechanism
The data binding mechanism is perhaps the most crucial aspect of an adapter's function. Essentially, it is the process through which adapters connect data to view components within an Android application. This process allows developers to keep the user interface in sync with the underlying data model.
- Flexibility: Different types of data sources can be managed using various adapters, like ArrayAdapter or CursorAdapter. Each adapter type is built to handle specific data structures, from simple lists to complex databases. This flexibility ensures that developers can choose the most effective tool for the job.
- Efficiency: When data sets are large, an efficient binding process is paramount. Adapters optimize memory usage and reduce view creation overhead, especially when implementing the ViewHolder pattern. This results in smoother scrolling and quicker UI updates, as only the visible items are bound.
- Real-Time Updates: A well-designed data binding mechanism can respond to changes in the data source dynamically. This is managed through callback functions or observers, which notify the adapter to refresh its views whenever the data is updated, keeping the UI responsive and in sync with the data.
The data binding mechanism ultimately strengthens the connection between a developer’s data and its representation within the user interface. Thus, understanding the nuances of this function is essential for enhancing both performance and user experience.
View Management
View management encompasses the various strategies employed by adapters to create, recycle, and update views dynamically. Effective view management is fundamental to optimizing performance in applications that display large lists or grids of data.
- View Creation and Recycling: Adapters are responsible for efficiently creating and recycling view objects. By implementing view recycling, an adapter can reuse view components rather than constantly inflating new ones from XML layouts. This significantly reduces the overhead associated with view creation.
- View Binding: Once a view is created or recycled, it must be bound to its corresponding data entry. This includes populating the view with text, images, or any other relevant information. This step must be performed efficiently to ensure that the UI updates quickly and without lag.
- User Interaction: Adapters also manage how views respond to user actions. For example, selecting an item in a list needs to trigger an event that can be handled by the application. This interaction capability is vital for maintaining a responsive and intuitive user experience.
In summary, the core functions of adapters—data binding and view management—play a pivotal role in enhancing the overall efficiency of Android applications. Understanding these functions not only aids developers in making informed design decisions but also allows for more efficient and responsive applications.
Types of Android Adapters
The topic of Android adapters is critical in understanding how data is managed and displayed within Android applications. Different types of adapters are designed for specific needs and scenarios, which can enhance the overall user experience and application performance. Each adapter type serves a unique purpose and has its advantages and disadvantages. IT professionals must comprehend these variations to effectively select the appropriate adapter for their requirements.
ArrayAdapter
ArrayAdapter is a widely utilized adapter in Android development, known for its simplicity and effectiveness in binding arrays to views. It takes an array of items or a list and converts each item into a view. One of its main advantages is its ease of use. Developers can quickly populate ListViews or Spinner widgets with a static list of data without heavy customizations. For example, if a developer wants to show a list of strings like names or cities, using ArrayAdapter is straightforward and efficient.
Example:
It’s important to note that while ArrayAdapter is effective for basic use cases, it may struggle with performance when handling large datasets. In such situations, other adapter types might be more suitable.
CursorAdapter
CursorAdapter holds an essential role when dealing with data from a SQLite database. It is specifically tailored to interact with a content provider or query a database, making it perfect for displaying data efficiently within views. CursorAdapters bind a Cursor object to a ListView or other AdapterViews, allowing for dynamic updates when the data source changes.
This type of adapter is optimal for applications that require real-time data updates. For instance, when a database is modified, the CursorAdapter automatically re-queries the database and updates the UI without requiring explicit data management from the developer.
Consideration:
- Managing cursors efficiently is crucial. Developers need to ensure that cursors are properly closed after use to avoid memory leaks.
SimpleAdapter
SimpleAdapter is another straightforward option. It allows developers to bind data from an array of maps to a ListView. Each map represents an item in the list and displays data dynamically. SimpleAdapter is beneficial when working with simple data structures like key-value pairs.
One of the key advantages is that it requires minimal setup. You can use it to display different data types, such as strings, images, or even custom layouts for individual list items. The flexibility it offers makes it a valuable choice in the right situations.
Usage Example:
BaseAdapter
BaseAdapter serves as a more customizable adapter option. Unlike ArrayAdapter and SimpleAdapter, BaseAdapter allows for more flexibility in managing complex layouts and datasets. Developers can create their implementation of the adapter by extending BaseAdapter, overriding its necessary methods such as , , and .
This provides fine-grained control over the performance and layout management. BaseAdapter is particularly useful for applications that require dynamic, complex, or custom views that default adapters cannot handle efficiently.
Key Benefits:
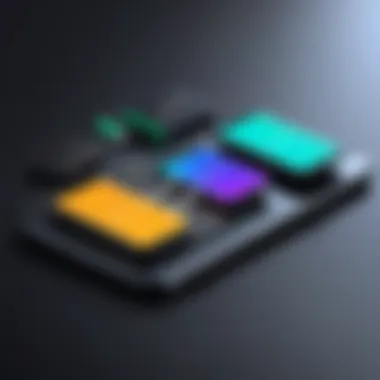
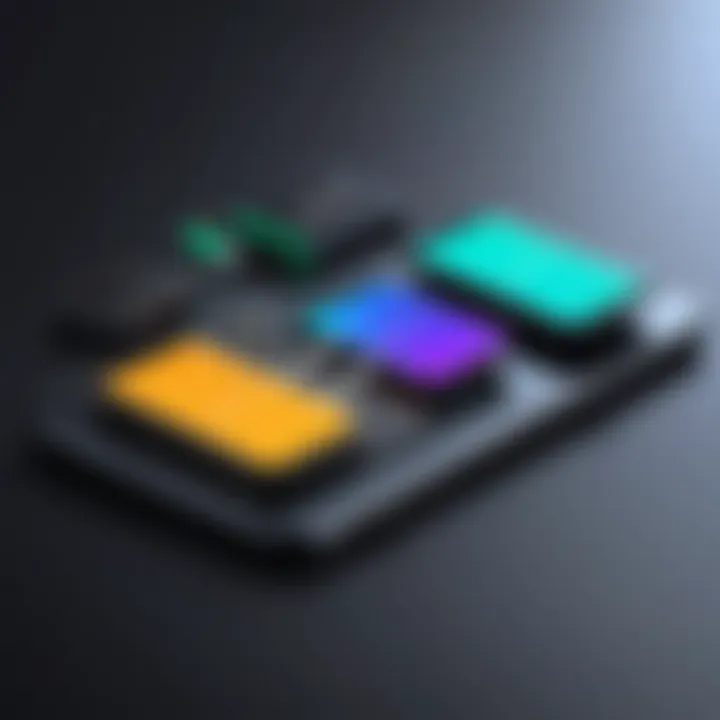
- Customization of layouts and view inflation.
- Performance improvements for large datasets through optimization.
- Control over item recycling and view types for varied layouts.
Creating a Custom Adapter
Creating a custom adapter is essential for tailoring the data presentation and behavior according to specific needs in an Android application. While built-in adapters such as ArrayAdapter and SimpleAdapter are useful, they often fall short when a unique presentation or interaction is required. Developing a custom adapter allows for greater flexibility, increased performance, and enhanced user experience. Without the constraints of predefined behaviors, developers can create more efficient and aesthetically pleasing user interfaces.
Extending BaseAdapter
To create a custom adapter, one primarily begins by extending the BaseAdapter class. This class provides a foundation for building adapter logic and promotes reusability across different parts of the application. By extending BaseAdapter, developers gain access to key abstract methods that need implementation. These include , , , and . Each method serves a purpose:
- returns the total number of items to be displayed.
- fetches the item at a specified position.
- returns the ID of the item at the specified position.
- is where the layout for each item is inflated, and is most critical in defining how data is displayed.
Overriding Required Methods
The next critical step is overriding the required methods mentioned earlier. Proper implementation of these methods ensures the adapter functions correctly and efficiently. The method should accurately reflect the number of items in the underlying data source.
In the method, inflation of custom layouts is necessary. This method also allows the customization of how each item appears. It is vital for ensuring that each view gets the proper data representation. Reuse of views through the parameter can enhance performance. Reusing views prevents excessive memory use and increases the smoothness of UI updates.
Managing ViewHolder Pattern
Finally, employing the ViewHolder pattern within the custom adapter provides a significant performance boost. This pattern caches the views, reducing the need for repeated calls to , which can be a costly operation. It leads to faster scrolling and a more responsive interface. The ViewHolder holds all the views that are to be displayed for a single row, making them easily accessible without searching through the view hierarchy multiple times.
Here is how the ViewHolder pattern can be implemented within the method:
The ViewHolder pattern is crucial for facilitating smooth performance in custom adapters, especially with large data sets.
In summary, creating a custom adapter empowers developers to craft unique and optimized user experiences within Android applications. The advantages of extending BaseAdapter, overriding required methods, and managing the ViewHolder pattern significantly enhance the app's performance and efficiency.
Implementing Adapters in Activities
When we talk about adapters in the Android framework, it is essential to understand their implementation within activities. Adapters serve as a bridge between data and user interface components, thus playing a critical role in creating a fluid user experience. Implementing adapters in activities facilitates the effective representation of data collections in UI elements like lists and grids. Moreover, it optimizes the interaction between the data model and the visual layout, which is crucial for any application.
Binding Data to Views
Binding data to views is a core functionality of Android adapters. This process involves linking the data set, often an array or list of objects, to the UI components that will display this information, such as TextViews and ImageViews in a RecyclerView. To effectively bind data, developers typically extend one of the adapter classes such as ArrayAdapter or BaseAdapter. By doing so, they can gain control over how each item in the data set is rendered in the UI.
When binding, the adapter’s method is called for each item, allowing the developer to specify how the data should be displayed. For example, consider the following pseudo-code:
This example illustrates the essential bind between data and UI; it showcases how each item's data (in this case, ) is assigned to the respective view. This method of binding enhances performance through view recycling and is fundamental for achieving smooth scrolling in a list.
Handling UI Updates
Handling UI updates is another vital aspect of implementing adapters. As the underlying data changes, whether through user interactions or external data sources, the UI must reflect these changes promptly. Thus, an efficient implementation requires the ability to refresh the view appropriately.
To manage UI updates, the adapter class often includes methods like , which signals that the data set has changed and that the view should reflect these updates. It is important to call this method after making changes to the data, for instance, when adding or removing items. Here is a simple illustration of how this might be managed:
Incorporating proper handling of UI updates enhances user engagement by ensuring that users are always viewing the most recent data. It also prevents issues like displaying outdated or incorrect information, which could lead to confusion and diminish the application’s reliability.
In summary, understanding how to implement adapters within activities is crucial for Android developers. Efficiently binding data to views and handling updates translates into a more responsive and user-centric experience, thereby enhancing the overall performance of an Android application.
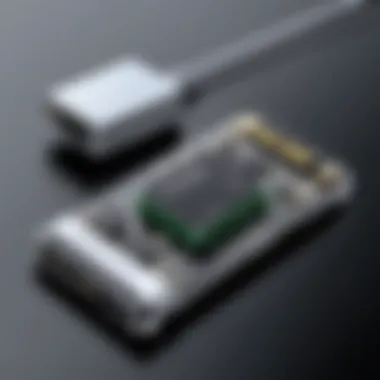
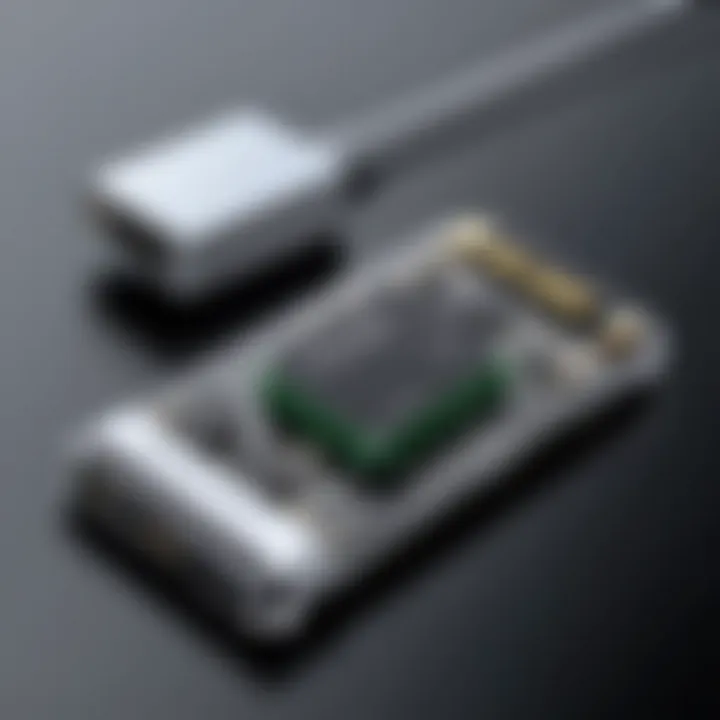
Best Practices for Using Adapters
When working with Android adapters, following best practices is crucial. These guidelines help ensure that the application runs efficiently and effectively. Poorly implemented adapters can lead to performance issues and a negative user experience. Thus, understanding best practices allows developers to create more optimized applications.
Performance Considerations
One of the key areas to focus on is performance. Android applications often face constraints, especially regarding resources and memory. Below are some practices that can significantly improve performance:
- Use ViewHolder Pattern: Implementing this pattern minimizes calls to , which can be resource-intensive. The ViewHolder stores references to the views in a layout. It reduces repetitive layout look-ups during scrolling.
- Recycle Views: Ensure that your adapter takes advantage of view recycling. This approach allows you to reuse views instead of inflating new ones every time they are needed. For example, does this automatically, significantly boosting performance.
- Limit Data Processing: Process data outside the main UI thread. Use background threads, such as or , to manage data fetching or processing. This prevents UI freezes and promotes a smooth user experience.
- Batch Updates: Instead of updating UI elements one at a time, group data updates into a single call. Using methods like is costly when called frequently. Instead, notify only after all changes are done, using and similar methods when possible.
By integrating these practices into your implementation, you can enhance responsiveness and fluidity in the user interaction.
Memory Management
Memory management is another essential aspect of using adapters. Efficient use of memory can prevent issues of sluggishness and facilitate better performance. Here are strategies to manage memory effectively:
- Avoid Holding References to Context: Storing a reference to an Activity or Fragment in the adapter can lead to memory leaks. Instead, use a weak reference or pass context only when necessary.
- Release Unused Resources: Make sure to clear any data or resources that are no longer needed when the adapter is no longer in use. This is especially important in the case of large images or data sets.
- Optimize Data Structure: Depending on the size of the data set, using more memory-efficient data structures can significantly improve performance. For instance, using instead of a regular array for scenarios involving sparse data can yield better memory utilization.
- Run Memory Profilers: Utilize Android Studio’s built-in tools to analyze memory usage. Memory leaks and high memory consumption can often go unnoticed but can significantly affect the user experience when overlooked.
Adopting these practices in memory management ensures that Android applications run smoothly and efficiently, delivering a satisfactory experience to users.
Common Issues and Troubleshooting
Understanding common issues that arise while working with Android adapters is essential for IT professionals and tech enthusiasts who aim to create robust applications. In real-world applications, even small mistakes can lead to significant problems that impact user experience. Addressing these concerns not only enhances the usability of the application but also improves developer productivity. This section will explore two common issues encountered when handling Android adapters: Null Pointer Exceptions and Inconsistent UI Data.
Null Pointer Exceptions
Null Pointer Exceptions are frequently encountered in Android development, particularly when dealing with adapters. This exception occurs when the code attempts to access an object that has not been initialized. In the context of an adapter, this can happen if a view or data source is null. For example, if an adapter tries to retrieve data from a list that has not been populated yet, a Null Pointer Exception will occur.
To avoid this issue, developers should implement proper null checks. Before accessing an object, always ensure that the object is instantiated. An example of this could be checking if the data list is not null before setting it to the adapter:
Additionally, using the ViewHolder pattern can minimize the risk of Null Pointer Exceptions by reducing the number of findViewById calls that may return null if a view does not exist.
Inconsistent UI Data
Inconsistent UI data is another common issue faced by developers using Android adapters. This issue arises when the UI does not reflect the underlying data model accurately. For instance, the displayed data may become outdated if the adapter does not notify the UI about changes in the data set. If data is modified after an adapter is set, the UI might not update accordingly, resulting in a confused user experience.
To mitigate this problem, it's crucial to use methods like notifyDataSetChanged() whenever the data set is updated. This method should be called after any insertions, deletions, or changes to the data so the adapter can re-query the data and refresh the UI, ensuring consistency. Here’s how to do it effectively:
By actively managing data updates, developers can ensure that the UI remains in sync with the data model, thereby enhancing user interaction.
In summary, understanding and troubleshooting these common issues is vital for successful implementation of Android adapters. Addressing Null Pointer Exceptions ensures stability in your application, while managing UI data consistency enhances overall user experience.
End
The conclusion serves as a vital component of this article, encapsulating the insights gathered throughout the discussion on Android adapters. It emphasizes the significance of understanding adapters, which is crucial for IT professionals and developers to create efficient mobile applications. By summarizing the core aspects, we reinforce the value of implementing the right adapter for specific scenarios, thereby improving user experience and optimizing performance.
Summary of Key Points
In reviewing the important elements discussed, we can highlight several key points:
- Definition and Role: Android adapters are integral for facilitating data binding to views and ensuring smooth interaction within the user interface.
- Types of Adapters: A variety of adapters are available, including ArrayAdapter, CursorAdapter, SimpleAdapter, and BaseAdapter, each tailored for specific data handling needs.
- Custom Adapters: The ability to develop custom adapters allows for personalization and enhanced functionality tailored to the application’s requirements.
- Implementation: Proper implementation strategies can significantly affect the overall user interaction and application performance.
- Common Issues: Knowing the potential pitfalls, such as Null Pointer Exceptions and inconsistent UI data, aids in proactivity and troubleshooting.
Future Trends in Android Adapters
Looking forward, we can expect several trends to shape the future of Android adapters:
- Increased Use of Jetpack Libraries: As developers lean towards modernization, libraries like Jetpack will enhance the efficiency of adapters with features such as Paging and LiveData integration.
- Focus on Performance Optimization: Continuous efforts to minimize memory usage and enhance responsiveness will lead to the evolution of new adapter types and methods.
- Improved Tools for Debugging and Testing: Enhanced tools for tracking adapter behavior and performance metrics will assist developers in identifying weaknesses and optimizing implementations.
- Adaptation to New UI Frameworks: With the emergence of new UI paradigms, such as Jetpack Compose, adapters will undergo transformations that better align with declarative programming models.
The thoughtful application of these trends will empower developers to further elevate user engagement in their applications. Understanding the mechanics and implications of Android adapters is not just beneficial; it is essential for crafting superior mobile experiences.